Feedback panel¶
Feedback panel provide easy to use on-screen instructions for hints and shortcuts.
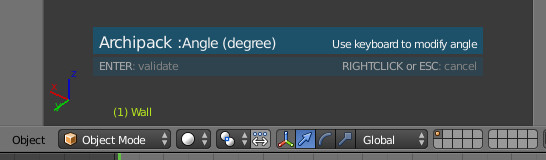
Feedback panel sample¶
Requirements¶
In order to use in your addon, you may copy archipack_gl.py
Archipack gl classes¶
archipack_gl provide some classes to draw either 2d or 3d (coords in pixels or world)
Lines
Arc
Text
Handles (tri and square)
Editable text
Cursor fence (like with B shorcut)
Cursor area (like when dragging with B shorcut)
FeedbackPanel
Note
FeedbackPanel interface will remain stable, while other parts currently need some cleanup and are likely to change. Use it as wrapper around opengl to allow easy update when gl Api change - benefit from archpack’s updates.
Implementation sample¶
import bpy
from bpy.types import Operator
from .archipack_gl import FeedbackPanel
class DEMO_OP_Feedback(Operator):
bl_idname = "demo.feedback"
bl_label = "Feedback"
bl_description = "Feedback panel in modal"
bl_options = {'REGISTER', 'UNDO'}
feedback = None
_handle = None
# draw callback
def draw_callback(self, _self, context):
self.feedback.draw(context)
def invoke(self, context, event):
# Create a panel instance
self.feedback = FeedbackPanel(title="Main Title")
self.feedback.instructions(context, "Sub title", "hint", [
('SHIFT', 'deselect'),
('CTRL', 'contains'),
('A', 'All'),
('I', 'Inverse'),
('ESC or RIGHTMOUSE', 'exit when done')
])
# Enable panel
self.feedback.enable()
# panel draw handler
args = (self, context)
self._handle = bpy.types.SpaceView3D.draw_handler_add(self.draw_callback, args, 'WINDOW', 'POST_PIXEL')
# add modal handler
context.window_manager.modal_handler_add(self)
return {'RUNNING_MODAL'}
def modal(self, context, event):
# tag area for gl redraw
context.area.tag_redraw()
# exit condition
if event.type in {'ESC', 'RIGHTMOUSE'}:
bpy.types.SpaceView3D.draw_handler_remove(self._handle, 'WINDOW')
self.feedback.disable()
return {'FINISHED'}
# do whatever you need
if user_do_something:
# here you are able to change feedback on the fly, calling instructions again
self.feedback.instructions(context, 'Title', 'Hints about usage', [
('SHORTCUT', 'Hints about shortcut'),
...
])
if no_feeddback_need_for_this_action:
# disable / enable feedback drawing at any time too
self.feedback.disable()
return {'RUNNING_MODAL'}